Introduction
.NET Multi-platform App UI (.NET MAUI) is a cross-platform framework for creating native mobile and desktop apps with C# and XAML.
Using .NET MAUI, you can develop apps that can run on Android, iOS, macOS, and Windows from a single shared code-base.
Who .NET MAUI is for
.NET MAUI is for developers who want to:
- Write cross-platform apps in XAML and C#, from a single shared code-base in Visual Studio.
- Share UI layout and design across platforms.
- Share code, tests, and business logic across platforms.
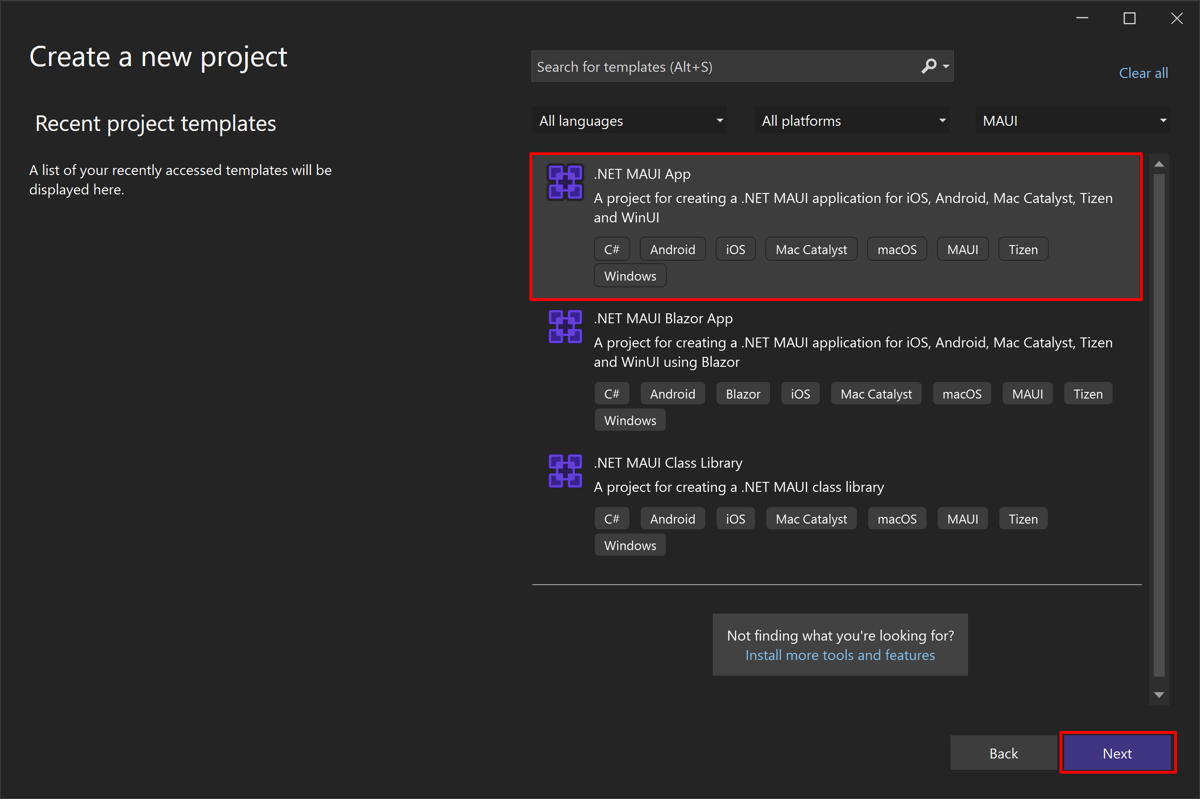
.NET MAUI stands for Multi-platform App UI, and it is a framework for building native mobile and desktop applications with a single shared code base.
As the successor to Xamarin Forms, .NET MAUI is the next major step in the evolution of Microsoft’s cross-platform development offering, allowing one to target Android, iOS, macOS, and Windows at the same time, thereby achieving even greater re-usability and development efficiency.
At Assemblysoft we specialise in designing and building high quality application solutions based on .NET, Azure, Blazor and .NET MAUI.
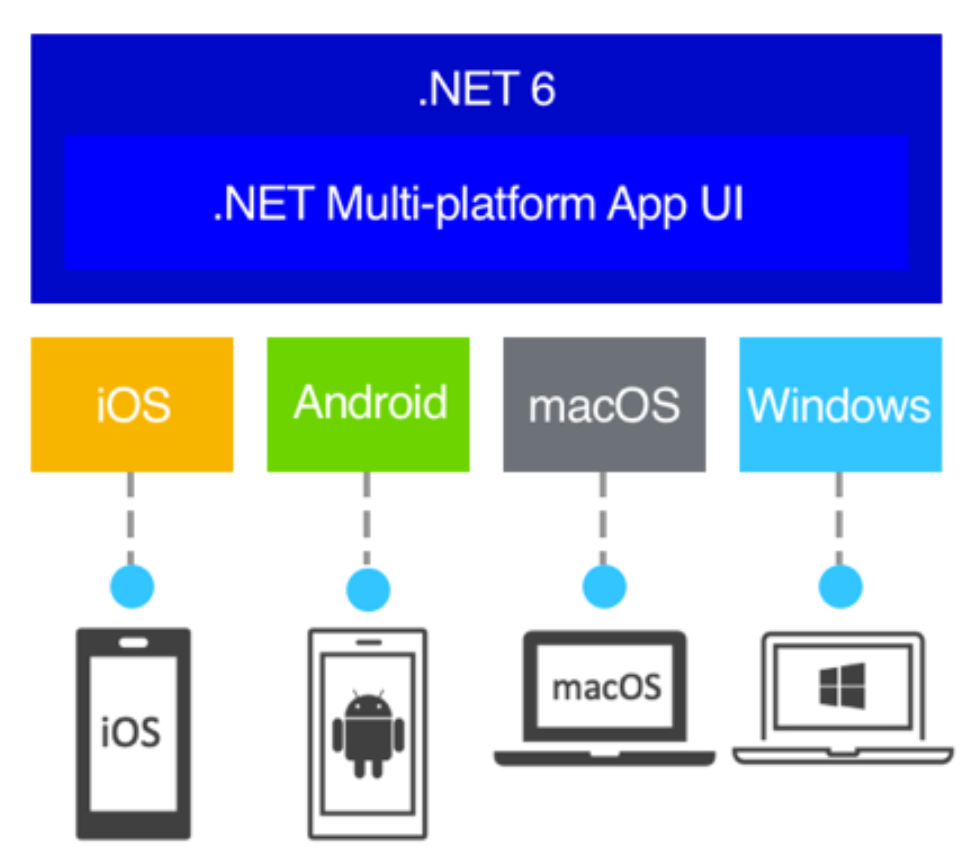
What .NET MAUI provides
.NET MAUI provides a collection of controls that can be used to display data, initiate actions, indicate activity, display collections, pick data, and more. In addition to a collection of controls, .NET MAUI also provides:
- An elaborate layout engine for designing pages.
- Multiple page types for creating rich navigation types, like drawers.
- Support for data-binding, for more elegant and maintainable development patterns.
- The ability to customize handlers to enhance the way in which UI elements are presented.
- Cross-platform APIs for accessing native device features. These APIs enable apps to access device features such as the GPS, the accelerometer, and battery and network states. For more information, see Cross-platform APIs for device features.
- Cross-platform graphics functionality, that provides a drawing canvas that supports drawing and painting shapes and images, compositing operations, and graphical object transforms.
- A single project system that uses multi-targeting to target Android, iOS, macOS, and Windows. For more information, see .NET MAUI Single project.
- .NET hot reload, so that you can modify both your XAML and your managed source code while the app is running, then observe the result of your modifications without rebuilding the app. For more information, see .NET hot reload.
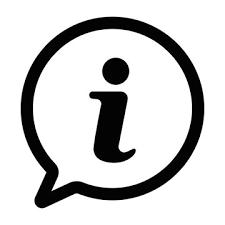
If you would like some assistance with .NET MAUI | Azure | Azure DevOps Services | Blazor Development then please get in touch, we would be glad to help.
Cross-platform APIs for device features
.NET MAUI provides cross-platform APIs for native device features. Examples of functionality provided by .NET MAUI for accessing device features includes:
- Access to sensors, such as the accelerometer, compass, and gyroscope on devices.
- Ability to check the device's network connectivity state, and detect changes.
- Provide information about the device the app is running on.
- Copy and paste text to the system clipboard, between apps.
- Pick single or multiple files from the device.
- Store data securely as key/value pairs.
- Utilize built-in text-to-speech engines to read text from the device.
- Initiate browser-based authentication flows that listen for a callback to a specific app registered URL.
Single project
.NET MAUI single project takes the platform-specific development experiences you typically encounter while developing apps and abstracts them into a single shared project that can target Android, iOS, macOS, and Windows.
.NET MAUI single project provides a simplified and consistent cross-platform development experience, regardless of the platforms being targeted. .NET MAUI single project provides the following features:
- A single shared project that can target Android, iOS, macOS, and Windows.
- A simplified debug target selection for running your .NET MAUI apps.
- Shared resource files within the single project.
- A single app manifest that specifies the app title, id, and version.
- Access to platform-specific APIs and tools when required.
- A single cross-platform app entry point.
.NET MAUI single project is enabled using multi-targeting and the use of SDK-style projects in .NET 6. For more information about .NET MAUI single project, see .NET MAUI single project.
Hot reload
.NET MAUI includes support for .NET hot reload, which enables you to modify your managed source code while the app is running, without the need to manually pause or hit a breakpoint. Then, your code edits can be applied to your running app without recompilation.
.NET MAUI includes support for XAML hot reload, which enables you to save your XAML files and see the changes reflected in your running app without recompilation. In addition, your navigation state and data will be maintained, enabling you to quickly iterate on your UI without losing your place in the app.
Platform-specific code
A .NET MAUI app project contains a Platforms folder, with each child folder representing a platform that .NET MAUI can target:
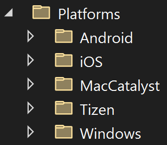
Multi-targeting can also be combined with conditional compilation so that code is targeted to specific platforms:
#if ANDROID
handler.NativeView.SetBackgroundColor(Colors.Red.ToNative());
#elif IOS
handler.NativeView.BackgroundColor = Colors.Red.ToNative();
handler.NativeView.BorderStyle = UIKit.UITextBorderStyle.Line;
#elif WINDOWS
handler.NativeView.Background = Colors.Red.ToNative();
#endif
NET Multi-platform App UI
Build native, cross-platform desktop and mobile apps all in one framework start reading the docs
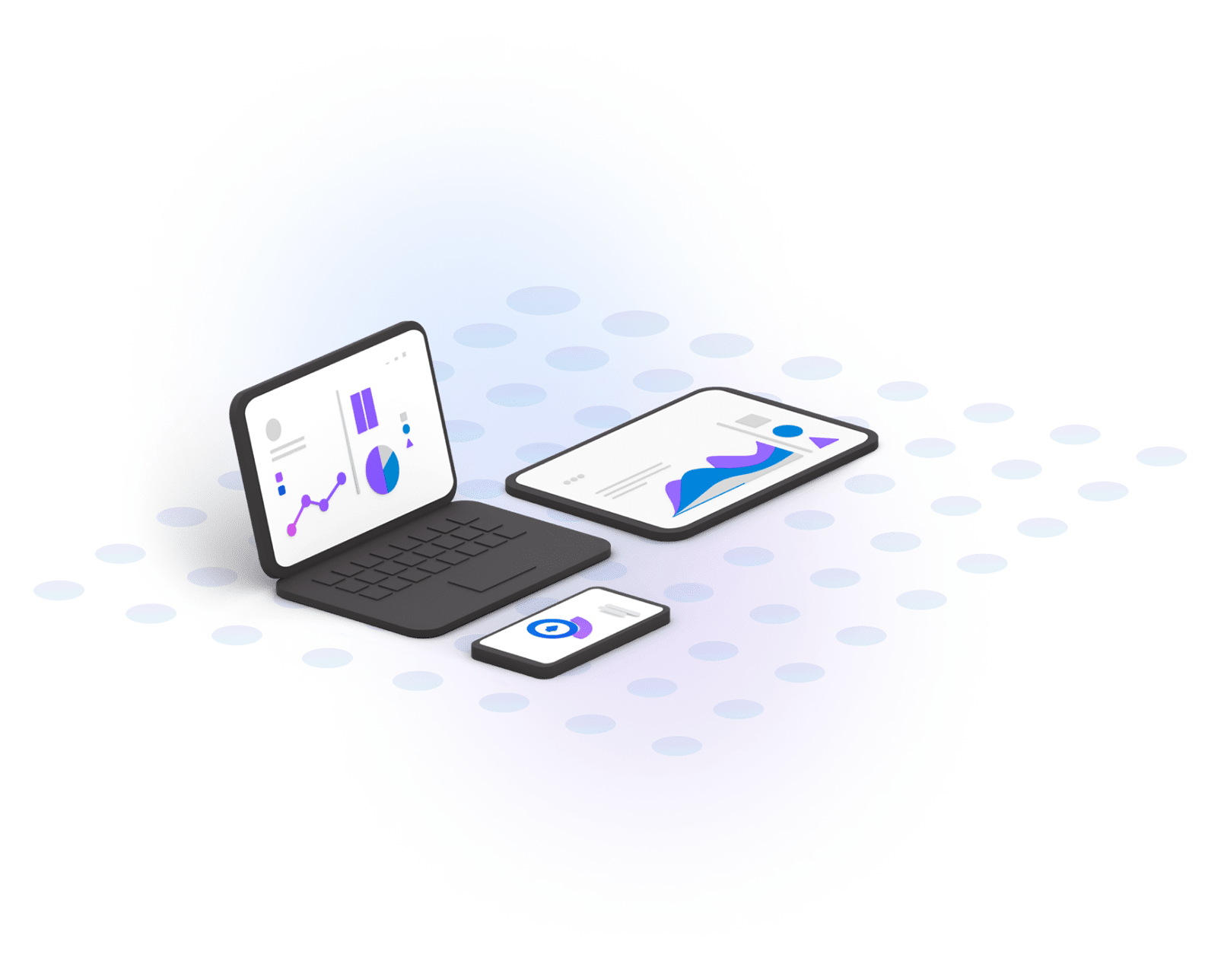
Multi-platform
.NET MAUI uses the latest technologies for building native apps on Windows, macOS, iOS, and Android, abstracting them into one common framework built on .NET.
One codebase
Use a single C# codebase and project system for all device targets to build apps that look and feel like the native platforms.
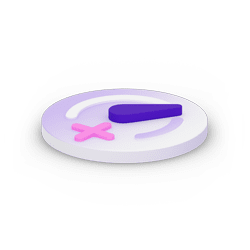
Productive
Build beautiful apps faster and easier by integrating the power of Visual Studio with .NET MAUI.
Supported platforms for .NET MAUI apps
.NET Multi-platform App UI (.NET MAUI) apps can be written for the following platforms:
- Android 5.0 (API 21) or higher.
- iOS 11 or higher, using the latest release of Xcode.
- macOS 10.15 or higher, using Mac Catalyst.
- Windows 11 and Windows 10 version 1809 or higher, using Windows UI Library (WinUI) 3.
.NET MAUI Blazor apps have the following additional platform requirements:
- Android 7.0 (API 24) or higher is required
- iOS 14 or higher is required.
- macOS 11 or higher, using Mac Catalyst.
Should you use .NET MAUI?
Whether you should be using .NET MAUI depends on your specific use case, and a few scenarios are discussed below.
You are already working with Xamarin Forms
Since MAUI is the successor to Xamarin Forms, offering many enhancements as discussed above, it makes perfect sense to upgrade your app to MAUI, the only question being around the timing of the upgrade.
You are planning to build a mobile app and are already invested in the .NET stack
If your company already has software built on .NET, whether for desktop or web, using MAUI for mobile app development would be a natural choice to:
- re-use common libraries and business logic,
- leverage the .NET knowledge within the team, and
- share UI code, either for an existing WinUI desktop application or by wrapping an existing Blazor-based web app into a MAUI container.
You are planning to build a cross-platform native mobile app but have not decided which technology to use
The three main players in the mobile cross-platform space for native apps are MAUI/Xamarin Forms, Flutter, and React Native.
One of the advantages that Flutter and Reactive Native had over Xamarin Forms was their fast inner-loop development experience allowing code changes to be visible immediately via a hot reload mechanism. .NET MAUI now provides full hot reload support for C# files as well, bringing it on par with Flutter and React Native.
You are planning to build a native cross-platform desktop app
If you only need to target Windows and macOS (via Mac Catalyst) with a cross-platform desktop app, then using MAUI can be a great choice because unlike many of the available cross-platform desktop frameworks, MAUI produces fully native apps, that have greater performance and OS integration compared to web UIs that are just hosted in a native container.
Currently, MAUI supports writing applications that run on Android 5+, iOS 10+, macOS 10.15+, Windows 10 version 1809+, or Windows 11. MAUI application project is a single . NET project.
Enhanced Developer Inner Loop with .NET MAUI
.NET MAUI addresses one of the pervasive challenges faced by mobile app developers: optimizing the inner loop, which pertains to swiftly reflecting markup or code changes on simulators/devices while the app is operational. The innovative feature Hot Reload paves the way for immediate visualization of code modifications on the simulator and devices, circumventing the necessity for full recompilation. Notably, it supports changes in .NET MAUI XAML visual tree markup and, in its future trajectory, will encompass most C# code modifications, boasting performance that rivals or even surpasses other cross-platform frameworks.
Revamped Toolkit for Enriched Developer Experience
Embracing the strengths and simplicity of .NET MAUI, the .NET MAUI Community Toolkit emerges as a crucial asset for developers. Available through two NuGet packages, CommunityToolkit.Maui and CommunityToolkit.Maui.Markup, the toolkit amplifies the .NET MAUI development experience. With a broad spectrum of Animations, Behaviors, Converters, and common UI Controls, along with fluent C# helper methods for crafting declarative user interfaces, it is ingeniously designed to provide comprehensive support to developers, ensuring a smooth, efficient development journey.
Seamless Migration and Modernization Capabilities
With .NET 6 possessing the LTS (Long-Term Support) badge, enterprises exploring migration and modernization avenues for their apps find a reliable ally in .NET MAUI. The framework provides fertile grounds to transplant and evolve existing desktop apps, enabling them to access platforms beyond Windows, while judiciously reusing a substantial portion of the existing code—provided the apps are well-architected. Furthermore, with the integration of Blazor Hybrid apps, developers are endowed with the substantial ability to share extensive code between native and web applications, ensuring cohesiveness and streamlined operations.
.NET MAUI offers a straightforward migration path for existing Xamarin.Forms apps, enabling a hassle-free transition using tools like the .NET Upgrade Assistant, and through activities like namespace remapping and dependency updating to .NET 6, thereby ensuring compatibility and performance are not compromised.
Ready and Robust UI Stack in .NET MAUI
.NET MAUI not only enters but enriches the .NET ecosystem with its adept capability to create cross-platform UIs with aplomb. While it ensures a repertoire of UI components similar to its predecessors, the community is dynamically engaged in bridging any existent gaps. Developers have the freedom and tools to select UI components that perfectly align with their projects/apps, and the Telerik UI for .NET MAUI provides a robust suite of controls that are worth exploring. Out-of-the-box UI components like ListViews, DataGrid, Charts, Gauges, Editors, and Input controls, which are integral for enterprise apps, ensure developers can expedite app delivery while maintaining the highest standards in UI design and functionality.
Exploring the Benefits of .NET MAUI
- Single Project for Multiple Platforms Manage all platform-related resources and configurations within a single project. This includes utilizing a singular application manifest, shared resource files, and a universal cross-platform entry point, streamlining development significantly.
- Enhanced Graphics APIs .NET MAUI provides a cross-platform graphics functionality and abstracts native drawing, offering a canvas for drawing and painting shapes and mitigating the need to manage drawings on the native side.
- Support for .NET 6 Leverage the powerful features of .NET 6 and C#10, integrating advancements and the latest functionalities directly into your development process with .NET MAUI.
- Utilization of Slim Renderers Adopt Slim Renderers to make applications more lightweight and enhance the development experience by streamlining rendering processes.
- Centralized Asset Management Manage all assets, including fonts, images, splash screen, and CSS, from a singular location, optimizing organizational efficiency.
- Efficient Platform-Specific Code Management With a dedicated "Platforms" folder, easily navigate and manage code specific to each supported platform, ensuring clear and organized codebase management.
- Support for MVU and Blazor Patterns .NET MAUI supports not only the Model-View-Update (MVU) pattern but also facilitates Blazor development patterns, providing flexible options for constructing cross-platform native front-ends.
- Blazor Support Enhancement .NET MAUI enriches Blazor's application, allowing it to be used in native desktop applications, enabling web developers to also deploy on desktop platforms with web-based rendering.
- Library Unification Integrating critical libraries such as Xamarin.Essentials, .NET MAUI allows for seamless usage of device capabilities and regular services like authentication and secure storage.
- Comprehensive Hot Reloads Support Modify XAML and managed source code while the application is running, and observe modifications without the need to stop, rebuild, or restart the application.
Additional Highlights of .NET MAUI
- Accessibility First Develop highly accessible applications with native UI, harnessing intrinsic accessibility support and providing a range of properties, such as description, hint, and heading level, to enhance user accessibility and experience.
- Platform Extensibility .NET MAUI is designed for extensibility, ensuring developers can customize elements like the Entry control with ease and efficiency, adapting to platform-specific rendering differences with just a few lines of code.
- Service Accessibility through APIs Easily customize controls and access native platform features, removing potential limitations and enhancing feature implementation with .NET MAUI's architectural design focused on extensibility.
- Global Using Statements & File-Scoped Namespaces With C# 10 features, .NET MAUI simplifies file management and reduces clutter, enhancing code readability and maintenance.
- Blazor for Desktop and Mobile Leverage your web development skills across platforms, executing Blazor components natively and rendering them to an embedded web view control, while also accessing native platform features like notifications, Bluetooth, and more.
With a myriad of benefits and advanced features, .NET MAUI paves the way for an elevated, streamlined, and efficient app development journey across various platforms. For more detailed information and resources, visit MAUI Documentation.
And of course, Blazor...
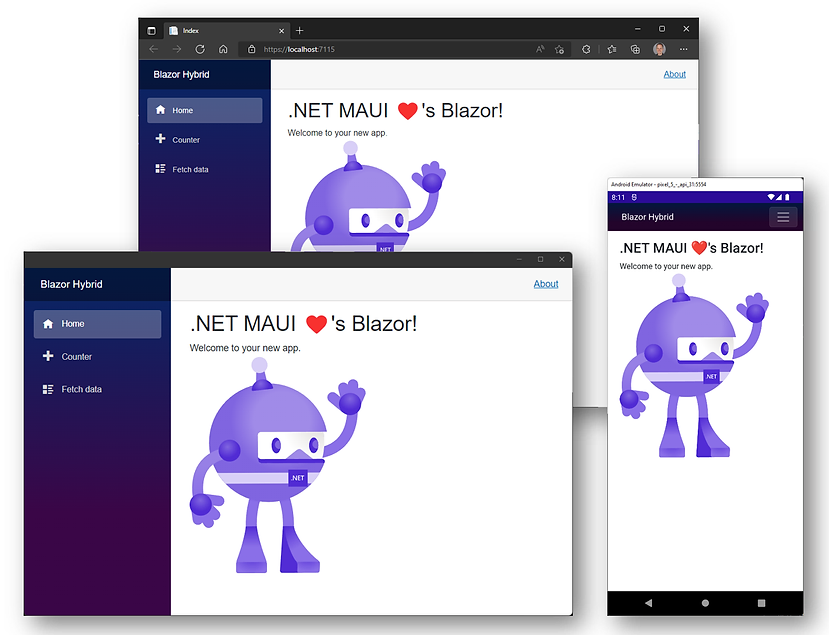
Assemblysoft has masterfully embraced Blazor, the revolutionary web framework which has garnered immense traction amongst .NET developers for its seamless integration of C# code, both on the client and server side, facilitated by Wasm. Recognizing the significant allure of the Blazor component model and the extensive enterprise investments in web technologies, Assemblysoft envisioned a synergy with native apps.
Harnessing the capabilities of .NET MAUI, Assemblysoft has crafted a number of applications that seamlessly amalgamate Blazor's prowess with MAUI's expansive reach across mobile and desktop platforms. At the heart of this integration is .NET MAUI's Blazor Hybrid apps framework. What this means in practice is that while .NET MAUI lays down the foundational app bootstrapping combined with comprehensive native API access, it further introduces the transformative BlazorWebView. This dynamic component can effortlessly accommodate an entire Blazor app or any other SPA-driven web application.
Conclusion
.NET MAUI’s key advantage is the mature, sophisticated, and widely available .NET ecosystem that provides great interoperability and code re-usability, high quality and well-supported libraries, powerful tools and IDEs, and a large active community of developers. Depending on what your business needs are, choosing the .NET stack is often a preferred option, especially for enterprise development, because of the stability, open-source, re-usability, support and longevity that it offers.
Our experience has been great and our developers absolutely love working with .NET MAUI. We would be happy to share our experiences with you, having now completed the development of a number of commercial, cross-platform applications using .NET MAUI targeting: IOS | Android | Windows. We are actively developing a number of Blazor Hybrid (.NET MAUI Blazor applications) from our Bournemouth based development agency in the United Kingdom.
Assemblysoft are a .NET Agency with a focus on .NET MAUI and Blazor
At Assemblysoft we specialise in Custom Software Development tailored to your requirements. We can onboard and add value to your business rapidly. We are an experienced Full-stack development team able to provide specific technical expertise or manage your project requirements end to end. We specialise in the Microsoft cloud and .NET Solutions and Services. Our developers are Microsoft Certified. We have real-world experience developing .NET applications and Azure Services for a large array of business domains. If you would like some assistance with Azure | Azure DevOps Services | Blazor Development | .NET MAUI Development or in need of custom software development, from an experienced development team in the United Kingdom, then please get in touch, we would love to add immediate value to your business.
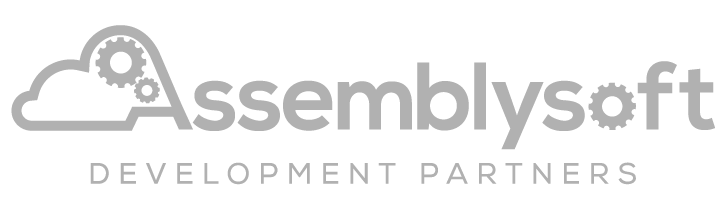
Assemblysoft - Your Safe Pair of Hands